Modularization in ABAP
Modularization is breaking down the application code into smaller units, so that it is easy to maintain. Suppose if we want to implement the same logic like adding two numbers in several places of the same program, then write the logic inside a modularization unit and call the modularization unit where ever we want to add two numbers.
Even if we want to declare the same variables in multiple programs or use the same logic in different programs then code the common part in the modularization unit and use it in different programs.
Advantages of Modularization are as follows.
If we want to reuse the same set of statements more than once in a program, we can include them in a macro. We can only use a macro within the program in which it is defined. Macro definition should occur before the macro is used in the program.
We can use the following syntax to define a macro.
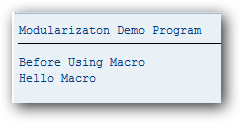
We can pass up to 9 placeholders to Macros.
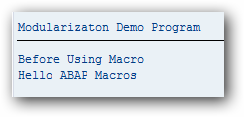
ABAP Include Program
Include programs are not standalone programs and cannot be executed independently. They can be used as a container for ABAP source code. They are used to organize the ABAP source code into small editable units which can be inserted at any place in other ABAP programs using the INCLUDE statement. Include programs can be used in different programs. The include statement copies the contents of the include program into the main program.
Include programs have no parameter interface and they cannot call themselves.
While creating the INCLUDE program using the ABAP editor choose program type as "I" i.e. INCLUDE program in the program attributes.

Syntax for Include program is as follows.

ABAP Subroutine
Subroutines are procedures that we define in an ABAP program and can be called from any program. Subroutines are normally called internally, i.e. called from the same program in which it is defined. But it is also possible to call a subroutine from an external program. Subroutines cannot be nested and are usually defined at the end of the program.
A subroutine can be defined using FORM and ENDFORM statements.
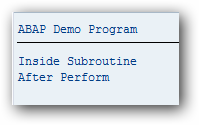
Subroutines can call other subroutines and may also call themselves. Once a subroutine has finished running, the control returns to the next statement after the PERFORM statement.
We can terminate a subroutine by using the EXIT or CHECK statement.
EXIT statement can be used to terminate a subroutine unconditionally. The control returns to the next statement after the PERFORM statement.

CHECK statement can be used to terminate a subroutine conditionally. If the logical expression in the CHECK statement is untrue, the subroutine is terminated, and the control returns to the next statement after the PERFORM statement.
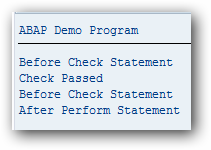
Modularization is breaking down the application code into smaller units, so that it is easy to maintain. Suppose if we want to implement the same logic like adding two numbers in several places of the same program, then write the logic inside a modularization unit and call the modularization unit where ever we want to add two numbers.
Even if we want to declare the same variables in multiple programs or use the same logic in different programs then code the common part in the modularization unit and use it in different programs.
Advantages of Modularization are as follows.
- Easier to read, maintain and debug.
- Eliminates redundancy of code and increases reusability of code .
- Macros – If you want to reuse the same set of statements more than once in a program, you can include them in a macro. You can only use a macro within the program in which it is defined.
- Include Programs – Include programs allow you to use the same source code in different programs. They are mainly used for modularizing source code and have no parameter interface.
- Subroutines – Subroutines are normally called internally i.e. called from the same program in which it is declared. But subroutines can also be called from external programs.
- Function Modules – Function Modules are stored in the central library and can be called from any program. Even the function modules(RFC enabled) can be called from non-SAP systems.
- Methods – Methods used ABAP object oriented programming
- Event Blocks – Event blocks begin with a event code and ends with next processing block.
- Dialog Modules – Dialog Modules are used in PBO and PAI events of Module Pool programs.
If we want to reuse the same set of statements more than once in a program, we can include them in a macro. We can only use a macro within the program in which it is defined. Macro definition should occur before the macro is used in the program.
We can use the following syntax to define a macro.
DEFINE <macro name>. ... END-OF-DEFINITION.Demo program using Macro.
*Macro definition DEFINE print. write:/ 'Hello Macro'. END-OF-DEFINITION. WRITE:/ 'Before Using Macro'. print.Output
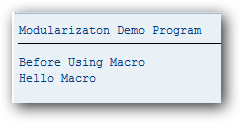
We can pass up to 9 placeholders to Macros.
*Macro definition DEFINE print. write:/ 'Hello', &1, &2. END-OF-DEFINITION. WRITE:/ 'Before Using Macro'. print 'ABAP' 'Macros'.Output
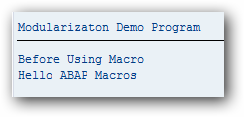
ABAP Include Program
Include programs are not standalone programs and cannot be executed independently. They can be used as a container for ABAP source code. They are used to organize the ABAP source code into small editable units which can be inserted at any place in other ABAP programs using the INCLUDE statement. Include programs can be used in different programs. The include statement copies the contents of the include program into the main program.
Include programs have no parameter interface and they cannot call themselves.
While creating the INCLUDE program using the ABAP editor choose program type as "I" i.e. INCLUDE program in the program attributes.

Syntax for Include program is as follows.
INCLUDE <Include Program Name>Source code of ZINCLUDE_DATA.
DATA: g_name(10) TYPE c.Source code of ZINCLUDE_WRITE.
WRITE:/ 'Inside include program'.Source code of main program.
REPORT zmain_program. INCLUDE zinclude_data. WRITE:/ 'Main Program'. INCLUDE zinclude_write.Output

ABAP Subroutine
Subroutines are procedures that we define in an ABAP program and can be called from any program. Subroutines are normally called internally, i.e. called from the same program in which it is defined. But it is also possible to call a subroutine from an external program. Subroutines cannot be nested and are usually defined at the end of the program.
A subroutine can be defined using FORM and ENDFORM statements.
FORM <subroutine name>. ... ENDFORM.A subroutine can be called using PERFORM statement.
PERFORM <subroutine name>.Example Program.
PERFORM sub_display.
WRITE:/ 'After Perform'.
*&---------------------------------------------------------------------*
*& Form sub_display
*&---------------------------------------------------------------------*
FORM sub_display.
WRITE:/ 'Inside Subroutine'.
ENDFORM. " sub_display
Output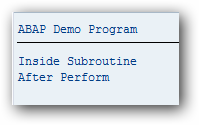
Subroutines can call other subroutines and may also call themselves. Once a subroutine has finished running, the control returns to the next statement after the PERFORM statement.
We can terminate a subroutine by using the EXIT or CHECK statement.
EXIT statement can be used to terminate a subroutine unconditionally. The control returns to the next statement after the PERFORM statement.
PERFORM sub_display. WRITE:/ 'After Perform Statement'. *&---------------------------------------------------------------------* *& Form sub_display *&---------------------------------------------------------------------* FORM sub_display. WRITE:/ 'Before Exit Statement'. EXIT. WRITE:/ 'After Exit Statement'. " This will not be executed ENDFORM. " sub_displayOutput

CHECK statement can be used to terminate a subroutine conditionally. If the logical expression in the CHECK statement is untrue, the subroutine is terminated, and the control returns to the next statement after the PERFORM statement.
DATA: flag TYPE c.
DO 2 TIMES.
PERFORM sub_display.
ENDDO.
WRITE:/ 'After Perform Statement'.
*&---------------------------------------------------------------------*
*& Form sub_display
*&---------------------------------------------------------------------*
FORM sub_display.
WRITE:/ 'Before Check Statement'.
CHECK flag NE 'X'.
WRITE:/ 'Check Passed'.
flag = 'X'.
ENDFORM. " sub_display
Output 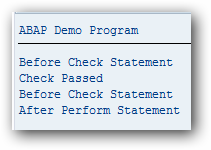
No comments:
Post a Comment