What is an ABAP Internal Table and How to Create it?
An Internal table is a temporary table gets created in the memory of application server during program execution and gets destroyed once the program ends. It is used to hold data temporarily or manipulate the data. It contains one or more rows with same structure.
An internal table can be defined using the keyword TABLE OF in the DATA statement. Internal table can be defined by the following ways.
Usually internal tables are used to hold data from database tables
temporarily for displaying on the screen or further processing. To fill
the internal table with database values, use SELECT statement to read
the records from the database one by one, place it in the work area and
then APPEND the values in the work area to internal table.
Using INTO TABLE addition to SELECT statement we can also read multiple records directly into the internal table directly. No work area used in this case. This select statement will not work in loop, so no ENDSELECT is required.
Inserting Lines into ABAP Internal Tables
We can insert one or more lines to ABAP internal tables using the INSERT statement. To insert a single line, first place the values we want to insert in a work area and use the INSERT statement to insert the values in the work area to internal table.
Syntax to insert a line to internal table
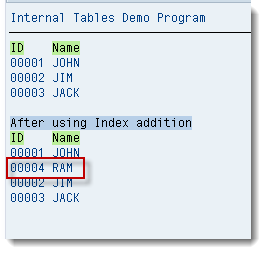
We can also insert multiple lines to an internal table with a single INSERT statement i.e. we can insert the lines of one internal table to another internal table.
Syntax to insert multiple lines to internal table
Output
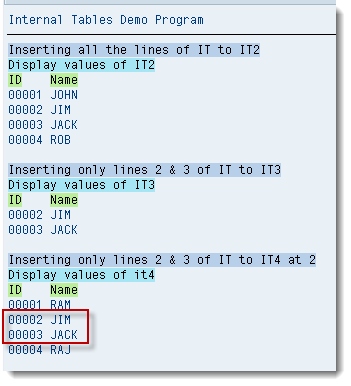
Deleting Lines from an ABAP Internal Table
DELETE is the statement to delete one or more lines from an ABAP Internal Table. Use the INDEX addition to delete a single line. If we use the INDEX addition and the operation is successful, SY-SUBRC will be set to zero, the line with the corresponding index in the internal table will be deleted and the indexes of the subsequent lines will be reduced by one.
We can use the WHERE clause to delete single or multiple lines. All the lines that meet the logical condition will be deleted. If at least one line is deleted, the system sets SY-SUBRC to 0, otherwise to 4.

More on ABAP Internal Tables
DESCRIBE TABLE is the statement to get the attributes like number of lines, line width of each row etc. of the internal table. DESCRIBE TABLE statement also fills the system fields SY-TFILL (Current no. of lines in internal table), SY-TLENG (line width of internal table) etc.

We can exit out of LOOP/ENDLOOP processing using EXIT, CONTINUE and CHECK similar to all other LOOPS.
We can also initialize the internal table using FREE, CLEAR and REFRESH statements. CLEAR and REFRESH just initializes the internal table where as FREE initializes the internal table and releases the memory space.
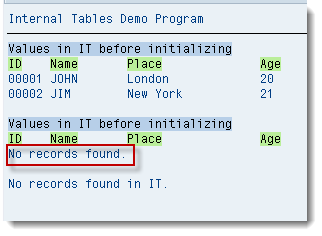
Control Break Processing in ABAP Internal Tables
Control break processing is used to execute a piece of code whenever a specific condition in the data is detected during the processing of internal table loop.
The following control break statements are available with in LOOP and ENDLOOP.
The code between AT LAST and ENDAT is executed only during the last loop pass. So it is used to write the totals or some report footers.
*————————————————————–* *Data Declaration *————————————————————–*

Between AT FIRST and ENDAT the work area will not contain any data. The default key fields are filled with asterisks(*) and the numeric fields are filled with zeros. The ENDAT restores the contents to the values they had prior to entering the AT FIRST. Changes to the work area within AT FIRST and ENDAT are lost. The same applies for AT LAST and ENDAT.
*————————————————————–* *Data Declaration *————————————————————–*

AT NEW and ENDAT is used to detect a change in the value of the field between the loop passes. The field that is specified in AT NEW is called control level. The code between AT NEW and ENDAT will be executed during the first loop pass and every time the value of the control level changes or any other field left to the control level changes. Between AT NEW and ENDAT all the fields in the work area that are right to the control level are filled with zeros and asterisks.
Similarly The code between AT END OF and ENDAT will be executed during the last loop pass and every time the value of the control level changes or any other field left to the control level changes.
*————————————————————–* *Data Declaration *————————————————————–*
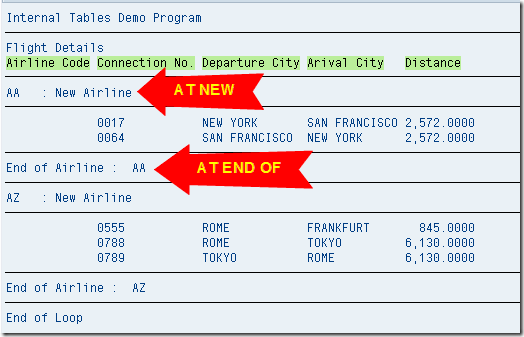
Control Break Processing in ABAP Internal Tables Continued
In AT FIRST and AT LAST event blocks the numeric values in the work area contains zeros. SUM statement calculates the totals of numeric fields and places the totals in the corresponding fields of work area.
In AT NEW and AT END OF event blocks SUM statement finds all the rows within the control level and calculates the totals of numeric fields that are right to the control level and places the totals in the corresponding fields of work area.
*————————————————————–* *Data Declaration *————————————————————–*
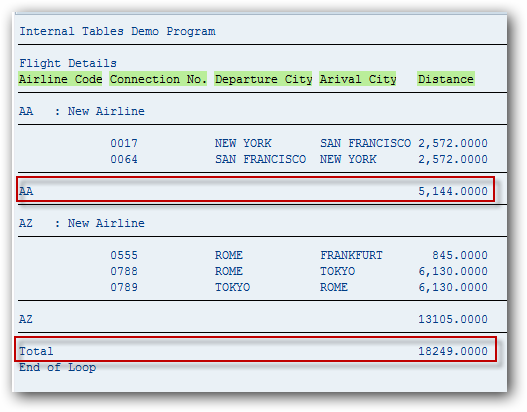
ON CHANGE OF behaves similar to AT NEW. The syntax is as follows.
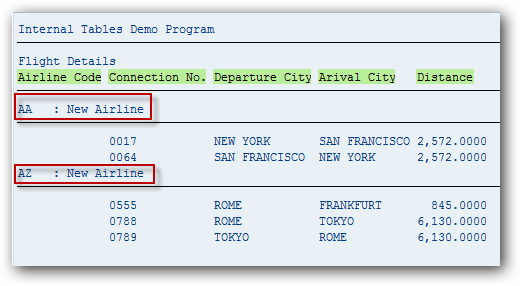
Below table summarizes the differences between AT NEW and ON CHANGE OF statements.
An Internal table is a temporary table gets created in the memory of application server during program execution and gets destroyed once the program ends. It is used to hold data temporarily or manipulate the data. It contains one or more rows with same structure.
An internal table can be defined using the keyword TABLE OF in the DATA statement. Internal table can be defined by the following ways.
TYPES: BEGIN OF ty_student,
id(5) TYPE n,
name(10) TYPE c,
END OF ty_student.
DATA: gwa_student TYPE ty_student.
"Referring to local data type
DATA: it1 TYPE TABLE OF ty_student.
"Referring to local data object
DATA: it2 LIKE TABLE OF gwa_student.
"Referring to data type in ABAP dictionary
DATA: it3 TYPE TABLE OF mara.
Use the APPEND statement to add data to internal table. First define
the work area i.e. define a field string with a structure similar to row
of the internal table. Then place the data in the work area and use the
APPEND statement to add the data from work area to internal table.*--------------------------------------------------------------* *Data Types *--------------------------------------------------------------* TYPES: BEGIN OF ty_student, id(5) TYPE n, name(10) TYPE c, END OF ty_student. DATA: gwa_student TYPE ty_student. *--------------------------------------------------------------* *Data Declaration *--------------------------------------------------------------* "Referring to local data type DATA: it TYPE TABLE OF ty_student. gwa_student-id = 1. gwa_student-name = 'JOHN'. APPEND gwa_student TO it. gwa_student-id = 2. gwa_student-name = 'JIM'. APPEND gwa_student TO it. gwa_student-id = 3. gwa_student-name = 'JACK'. APPEND gwa_student TO it.After the last APPEND statement in the above program, internal table ‘IT’ has the following 3 entries. But the internal table values are not persistent i.e. the internal table and its values are discarded once the program ends.
ID | Name |
---|---|
1 | JOHN |
2 | JIM |
3 | JACK |
DATA: gwa_employee TYPE zemployee,
gt_employee TYPE TABLE OF zemployee.
SELECT * FROM zemployee INTO gwa_employee.
APPEND gwa_employee TO gt_employee.
ENDSELECT.
After ENDSELECT the internal table GT_EMPLOYEE contains all the records that are present in table ZEMPLOYEE.Using INTO TABLE addition to SELECT statement we can also read multiple records directly into the internal table directly. No work area used in this case. This select statement will not work in loop, so no ENDSELECT is required.
SELECT * FROM zemployee INTO TABLE gt_employee.
Inserting Lines into ABAP Internal Tables
We can insert one or more lines to ABAP internal tables using the INSERT statement. To insert a single line, first place the values we want to insert in a work area and use the INSERT statement to insert the values in the work area to internal table.
Syntax to insert a line to internal table
INSERT <work area> INTO TABLE <internal table>. OR INSERT <work area> INTO <internal table> INDEX <index>.The first INSERT statement without INDEX addition will simply add the record to the end of the internal table. But if we want to insert the line to specific location i.e. if we want to insert it as second record then we need to specify 2 as the index in the INSERT statement.
*--------------------------------------------------------------* *Data Types *--------------------------------------------------------------* TYPES: BEGIN OF ty_student, id(5) TYPE n, name(10) TYPE c, END OF ty_student. *--------------------------------------------------------------* *Data Declaration *--------------------------------------------------------------* DATA: gwa_student TYPE ty_student. DATA: it TYPE TABLE OF ty_student. gwa_student-id = 1. gwa_student-name = 'JOHN'. INSERT gwa_student INTO TABLE it. gwa_student-id = 2. gwa_student-name = 'JIM'. INSERT gwa_student INTO TABLE it. gwa_student-id = 3. gwa_student-name = 'JACK'. INSERT gwa_student INTO TABLE it. WRITE:/ 'ID' COLOR 5,7 'Name' COLOR 5. LOOP AT it INTO gwa_student. WRITE:/ gwa_student-id, gwa_student-name. ENDLOOP. SKIP. WRITE:/ 'After using Index addition' COLOR 4. gwa_student-id = 4. gwa_student-name = 'RAM'. INSERT gwa_student INTO it INDEX 2. WRITE:/ 'ID' COLOR 5,7 'Name' COLOR 5. LOOP AT it INTO gwa_student. WRITE:/ gwa_student-id, gwa_student-name. ENDLOOP.Output
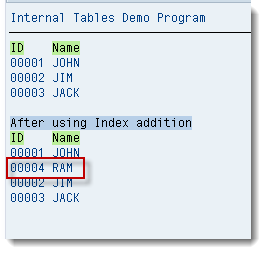
We can also insert multiple lines to an internal table with a single INSERT statement i.e. we can insert the lines of one internal table to another internal table.
Syntax to insert multiple lines to internal table
INSERT LINES OF <itab1> [FROM <index 1>] [TO <index 2>] INTO TABLE <itab2>. OR INSERT LINES OF <itab1> [FROM <index 1>] [TO <index 2>] INTO <itab2> INDEX <index>.
*--------------------------------------------------------------* *Data Types *--------------------------------------------------------------* TYPES: BEGIN OF ty_student, id(5) TYPE n, name(10) TYPE c, END OF ty_student. *--------------------------------------------------------------* *Data Declaration *--------------------------------------------------------------* DATA: gwa_student TYPE ty_student. DATA: it TYPE TABLE OF ty_student, it2 TYPE TABLE OF ty_student, it3 TYPE TABLE OF ty_student, it4 TYPE TABLE OF ty_student. gwa_student-id = 1. gwa_student-name = 'JOHN'. INSERT gwa_student INTO TABLE it. gwa_student-id = 2. gwa_student-name = 'JIM'. INSERT gwa_student INTO TABLE it. gwa_student-id = 3. gwa_student-name = 'JACK'. INSERT gwa_student INTO TABLE it. gwa_student-id = 4. gwa_student-name = 'ROB'. INSERT gwa_student INTO TABLE it. WRITE:/ 'Inserting all the lines of IT to IT2' COLOR 4. INSERT LINES OF it INTO TABLE it2. WRITE:/ 'Display values of IT2' COLOR 1. WRITE:/ 'ID' COLOR 5,7 'Name' COLOR 5. LOOP AT it2 INTO gwa_student. WRITE:/ gwa_student-id, gwa_student-name. ENDLOOP. SKIP. WRITE:/ 'Inserting only lines 2 & 3 of IT to IT3' COLOR 4. INSERT LINES OF it FROM 2 TO 3 INTO TABLE it3. WRITE:/ 'Display values of IT3' COLOR 1. WRITE:/ 'ID' COLOR 5,7 'Name' COLOR 5. LOOP AT it3 INTO gwa_student. WRITE:/ gwa_student-id, gwa_student-name. ENDLOOP. gwa_student-id = 1. gwa_student-name = 'RAM'. INSERT gwa_student INTO TABLE it4. gwa_student-id = 4. gwa_student-name = 'RAJ'. INSERT gwa_student INTO TABLE it4. SKIP. WRITE:/ 'Inserting only lines 2 & 3 of IT to IT4 at 2' COLOR 4. INSERT LINES OF it FROM 2 TO 3 INTO it4 INDEX 2. WRITE:/ 'Display values of it4' COLOR 1. WRITE:/ 'ID' COLOR 5,7 'Name' COLOR 5. LOOP AT it4 INTO gwa_student. WRITE:/ gwa_student-id, gwa_student-name. ENDLOOP.The last INSERT statement in the above program inserts the 2nd and 3rd line from IT at index 2 in IT4, so the new lines inserted becomes the 2nd and 3rd line in IT4.
Output
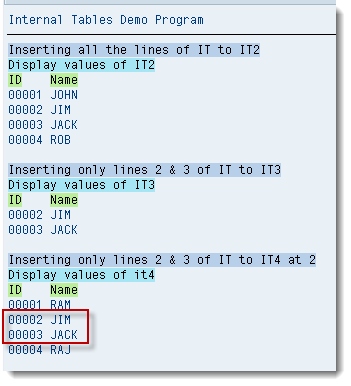
Deleting Lines from an ABAP Internal Table
DELETE is the statement to delete one or more lines from an ABAP Internal Table. Use the INDEX addition to delete a single line. If we use the INDEX addition and the operation is successful, SY-SUBRC will be set to zero, the line with the corresponding index in the internal table will be deleted and the indexes of the subsequent lines will be reduced by one.
DELETE <internal table> [INDEX <index>].We can also use the above DELETE statement without INDEX addition inside LOOP. Inside LOOP if we do not specify the INDEX, then the current loop line will be deleted.
We can use the WHERE clause to delete single or multiple lines. All the lines that meet the logical condition will be deleted. If at least one line is deleted, the system sets SY-SUBRC to 0, otherwise to 4.
DELETE <internal table> [FROM <n1>] [TO <n2>] [WHERE <condition>].With WHERE clause we can also specify the lines between certain indices that we want to delete by specifying indexes in FROM and TO additions.
*--------------------------------------------------------------* *Data Types *--------------------------------------------------------------* TYPES: BEGIN OF ty_student, id(5) TYPE n, name(10) TYPE c, place(10) TYPE c, age TYPE i, END OF ty_student. *--------------------------------------------------------------* *Data Declaration *--------------------------------------------------------------* DATA: gwa_student TYPE ty_student. DATA: it TYPE TABLE OF ty_student. gwa_student-id = 1. gwa_student-name = 'JOHN'. gwa_student-place = 'London'. gwa_student-age = 20. INSERT gwa_student INTO TABLE it. gwa_student-id = 2. gwa_student-name = 'JIM'. gwa_student-place = 'New York'. gwa_student-age = 21. INSERT gwa_student INTO TABLE it. gwa_student-id = 3. gwa_student-name = 'JACK'. gwa_student-place = 'Bangalore'. gwa_student-age = 20. INSERT gwa_student INTO TABLE it. gwa_student-id = 4. gwa_student-name = 'ROB'. gwa_student-place = 'Bangalore'. gwa_student-age = 22. INSERT gwa_student INTO TABLE it. WRITE:/ 'Values in IT before DELETE' COLOR 4. WRITE:/ 'ID' COLOR 5,7 'Name' COLOR 5, 18 'Place' COLOR 5, 37 'Age' COLOR 5. LOOP AT it INTO gwa_student. WRITE:/ gwa_student-id, gwa_student-name, gwa_student-place, gwa_student-age. ENDLOOP. SKIP. WRITE:/ 'Values in IT after DELETE' COLOR 4. *Delete second line from IT DELETE it INDEX 2. WRITE:/ 'ID' COLOR 5,7 'Name' COLOR 5, 18 'Place' COLOR 5, 37 'Age' COLOR 5. LOOP AT it INTO gwa_student. WRITE:/ gwa_student-id, gwa_student-name, gwa_student-place, gwa_student-age. ENDLOOP. SKIP. WRITE:/ 'Values in IT after DELETE using WHERE Clause' COLOR 4. *Delete entries from IT where place is Bangalore DELETE it WHERE place = 'Bangalore'. WRITE:/ 'ID' COLOR 5,7 'Name' COLOR 5, 18 'Place' COLOR 5, 37 'Age' COLOR 5. LOOP AT it INTO gwa_student. WRITE:/ gwa_student-id, gwa_student-name, gwa_student-place, gwa_student-age. ENDLOOP.Output

More on ABAP Internal Tables
DESCRIBE TABLE is the statement to get the attributes like number of lines, line width of each row etc. of the internal table. DESCRIBE TABLE statement also fills the system fields SY-TFILL (Current no. of lines in internal table), SY-TLENG (line width of internal table) etc.
DESCRIBE TABLE <internal table> [LINES <lines>].SORT is the statement to sort an ABAP internal table. We can specify the direction of the sort using the additions ASCENDING and DESCENDING. The default is ascending.
SORT <internal table> [ASCENDING|DESCENDING]We can also delete the adjacent duplicates from an internal table by using the following statement.
DELETE ADJACENT DUPLICATE ENTRIES FROM <internal table> [COMPARING <f1> <f2> ... |ALL FIELDS].COMPARING ALL FIELDS is the default. If we do not specify the COMPARING addition, then the system compares all the fields of both the lines. If we specify fields in the COMPARING clause, then the system compares only the fields specified after COMPARING of both the lines. If at least one line is deleted, the system sets SY-SUBRC to 0, otherwise to 4.
*--------------------------------------------------------------* *Data Types *--------------------------------------------------------------* TYPES: BEGIN OF ty_student, id(5) TYPE n, name(10) TYPE c, place(10) TYPE c, age TYPE i, END OF ty_student. *--------------------------------------------------------------* *Data Declaration *--------------------------------------------------------------* DATA: gwa_student TYPE ty_student. DATA: it TYPE TABLE OF ty_student. DATA: gv_lines TYPE i. gwa_student-id = 1. gwa_student-name = 'JOHN'. gwa_student-place = 'London'. gwa_student-age = 20. APPEND gwa_student TO it. gwa_student-id = 2. gwa_student-name = 'JIM'. gwa_student-place = 'New York'. gwa_student-age = 21. APPEND gwa_student TO it. gwa_student-id = 3. gwa_student-name = 'JACK'. gwa_student-place = 'Bangalore'. gwa_student-age = 20. APPEND gwa_student TO it. gwa_student-id = 4. gwa_student-name = 'ROB'. gwa_student-place = 'Bangalore'. gwa_student-age = 22. APPEND gwa_student TO it. gwa_student-id = 2. gwa_student-name = 'JIM'. gwa_student-place = 'New York'. gwa_student-age = 21. APPEND gwa_student TO it. DESCRIBE TABLE it LINES gv_lines. WRITE:/ 'No. of lines in IT : ', gv_lines. WRITE:/ 'SY-TFILL : ', sy-tfill. WRITE:/ 'SY-TLENG : ', sy-tleng. WRITE:/ 'Values in IT before SORT' COLOR 4. WRITE:/ 'ID' COLOR 5,7 'Name' COLOR 5, 18 'Place' COLOR 5, 37 'Age' COLOR 5. LOOP AT it INTO gwa_student. WRITE:/ gwa_student-id, gwa_student-name, gwa_student-place, gwa_student-age. ENDLOOP. WRITE:/ 'Values in IT after SORT' COLOR 4. *SORT by name SORT it BY name DESCENDING. WRITE:/ 'ID' COLOR 5,7 'Name' COLOR 5, 18 'Place' COLOR 5, 37 'Age' COLOR 5. LOOP AT it INTO gwa_student. WRITE:/ gwa_student-id, gwa_student-name, gwa_student-place, gwa_student-age. ENDLOOP. WRITE:/ 'Values in IT after deleting duplicates' COLOR 4. *Delete duplicates SORT it. DELETE ADJACENT DUPLICATES FROM it. WRITE:/ 'ID' COLOR 5,7 'Name' COLOR 5, 18 'Place' COLOR 5, 37 'Age' COLOR 5. LOOP AT it INTO gwa_student. WRITE:/ gwa_student-id, gwa_student-name, gwa_student-place, gwa_student-age. ENDLOOP. WRITE:/ 'Values in IT after deleting duplicates comparing place' COLOR 4. *Delete duplicates comparing only place SORT it BY place. DELETE ADJACENT DUPLICATES FROM it COMPARING place. WRITE:/ 'ID' COLOR 5,7 'Name' COLOR 5, 18 'Place' COLOR 5, 37 'Age' COLOR 5. LOOP AT it INTO gwa_student. WRITE:/ gwa_student-id, gwa_student-name, gwa_student-place, gwa_student-age. ENDLOOP.Output

We can exit out of LOOP/ENDLOOP processing using EXIT, CONTINUE and CHECK similar to all other LOOPS.
We can also initialize the internal table using FREE, CLEAR and REFRESH statements. CLEAR and REFRESH just initializes the internal table where as FREE initializes the internal table and releases the memory space.
*--------------------------------------------------------------* *Data Types *--------------------------------------------------------------* TYPES: BEGIN OF ty_student, id(5) TYPE n, name(10) TYPE c, place(10) TYPE c, age TYPE i, END OF ty_student. *--------------------------------------------------------------* *Data Declaration *--------------------------------------------------------------* DATA: gwa_student TYPE ty_student. DATA: it TYPE TABLE OF ty_student. DATA: gv_lines TYPE i. gwa_student-id = 1. gwa_student-name = 'JOHN'. gwa_student-place = 'London'. gwa_student-age = 20. APPEND gwa_student TO it. gwa_student-id = 2. gwa_student-name = 'JIM'. gwa_student-place = 'New York'. gwa_student-age = 21. APPEND gwa_student TO it. WRITE:/ 'Values in IT before initializing' COLOR 4. WRITE:/ 'ID' COLOR 5,7 'Name' COLOR 5, 18 'Place' COLOR 5, 37 'Age' COLOR 5. LOOP AT it INTO gwa_student. WRITE:/ gwa_student-id, gwa_student-name, gwa_student-place, gwa_student-age. ENDLOOP. *Initialize IT CLEAR it. SKIP. WRITE:/ 'Values in IT before initializing' COLOR 4. WRITE:/ 'ID' COLOR 5,7 'Name' COLOR 5, 18 'Place' COLOR 5, 37 'Age' COLOR 5. LOOP AT it INTO gwa_student. WRITE:/ gwa_student-id, gwa_student-name, gwa_student-place, gwa_student-age. ENDLOOP. *If no records are processed inside LOOP, then SY-SUBRC <> 0 IF sy-subrc <> 0. WRITE:/ 'No records found.'. ENDIF. SKIP. *We can also use IS INITIAL to check any records found in IT IF it IS INITIAL. WRITE:/ 'No records found in IT.'. ENDIF.Output
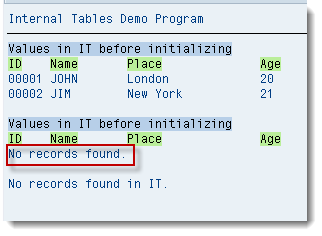
Control Break Processing in ABAP Internal Tables
Control break processing is used to execute a piece of code whenever a specific condition in the data is detected during the processing of internal table loop.
The following control break statements are available with in LOOP and ENDLOOP.
- AT FIRST / ENDAT
- AT LAST / ENDAT
- AT NEW / ENDAT
- AT END OF / ENDAT
- SUM
- ON CHANGE OF / ENDON
The code between AT LAST and ENDAT is executed only during the last loop pass. So it is used to write the totals or some report footers.
*————————————————————–* *Data Declaration *————————————————————–*
DATA: gwa_spfli TYPE spfli. DATA: gt_spfli TYPE TABLE OF spfli.*UP TO 5 ROWS addition selects only 5 rows from table SPFLI
SELECT * UP TO 5 ROWS FROM spfli INTO TABLE gt_spfli. LOOP AT gt_spfli INTO gwa_spfli.AT FIRST.
WRITE:/ 'Start of Loop'. WRITE:/ 'Flight Details'. WRITE:/ 'Airline Code' COLOR 5,14 'Connection No.' COLOR 5, 29 'Departure City' COLOR 5, 44 'Arival City' COLOR 5. ULINE.ENDAT.
WRITE:/ gwa_spfli-carrid,14 gwa_spfli-connid, 29 gwa_spfli-cityfrom,44 gwa_spfli-cityto.AT LAST.
ULINE. WRITE:/ 'End of Loop'.ENDAT.
ENDLOOP.Output

Between AT FIRST and ENDAT the work area will not contain any data. The default key fields are filled with asterisks(*) and the numeric fields are filled with zeros. The ENDAT restores the contents to the values they had prior to entering the AT FIRST. Changes to the work area within AT FIRST and ENDAT are lost. The same applies for AT LAST and ENDAT.
*————————————————————–* *Data Declaration *————————————————————–*
DATA: gwa_spfli TYPE spfli. DATA: gt_spfli TYPE TABLE OF spfli.*UP TO 5 ROWS addition selects only 5 rows from table SPFLI
SELECT * UP TO 5 ROWS FROM spfli INTO TABLE gt_spfli. LOOP AT gt_spfli INTO gwa_spfli. AT FIRST. WRITE:/ 'Flight Details'. WRITE:/ 'Airline Code' COLOR 5,14 'Connection No.' COLOR 5, 29 'Departure City' COLOR 5, 44 'Arival City' COLOR 5, 58 'Distance' COLOR 5.WRITE:/ gwa_spfli-carrid,14 gwa_spfli-connid, 29 gwa_spfli-cityfrom,44 gwa_spfli-cityto, 58 gwa_spfli-distance.
ULINE. ENDAT. WRITE:/ gwa_spfli-carrid,14 gwa_spfli-connid, 29 gwa_spfli-cityfrom,44 gwa_spfli-cityto, 58 gwa_spfli-distance. AT LAST. ULINE.WRITE:/ gwa_spfli-carrid,14 gwa_spfli-connid, 29 gwa_spfli-cityfrom,44 gwa_spfli-cityto, 58 gwa_spfli-distance.
WRITE:/ 'End of Loop'. ENDAT. ENDLOOP.Output

AT NEW and ENDAT is used to detect a change in the value of the field between the loop passes. The field that is specified in AT NEW is called control level. The code between AT NEW and ENDAT will be executed during the first loop pass and every time the value of the control level changes or any other field left to the control level changes. Between AT NEW and ENDAT all the fields in the work area that are right to the control level are filled with zeros and asterisks.
Similarly The code between AT END OF and ENDAT will be executed during the last loop pass and every time the value of the control level changes or any other field left to the control level changes.
*————————————————————–* *Data Declaration *————————————————————–*
DATA: gwa_spfli TYPE spfli. DATA: gt_spfli TYPE TABLE OF spfli. SELECT * UP TO 5 ROWS FROM spfli INTO TABLE gt_spfli. LOOP AT gt_spfli INTO gwa_spfli. AT FIRST. WRITE:/ 'Flight Details'. WRITE:/ 'Airline Code' COLOR 5,14 'Connection No.' COLOR 5, 29 'Departure City' COLOR 5, 44 'Arival City' COLOR 5, 58 'Distance' COLOR 5. ULINE. ENDAT.AT NEW carrid. WRITE:/ gwa_spfli-carrid, ‘ : New Airline’. ULINE. ENDAT.
WRITE:/14 gwa_spfli-connid, 29 gwa_spfli-cityfrom,44 gwa_spfli-cityto, 58 gwa_spfli-distance.AT END OF carrid. ULINE. WRITE:/ ‘End of Airline : ‘, gwa_spfli-carrid. ULINE. ENDAT.
AT LAST. WRITE:/ 'End of Loop'. ENDAT. ENDLOOP.Output
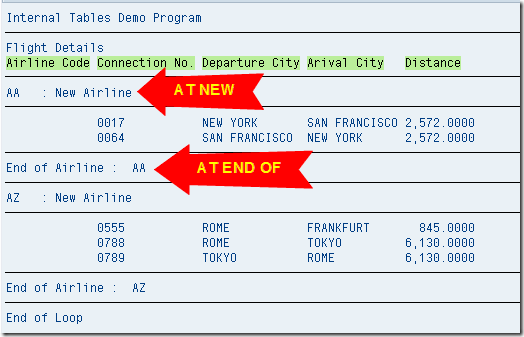
Control Break Processing in ABAP Internal Tables Continued
In AT FIRST and AT LAST event blocks the numeric values in the work area contains zeros. SUM statement calculates the totals of numeric fields and places the totals in the corresponding fields of work area.
In AT NEW and AT END OF event blocks SUM statement finds all the rows within the control level and calculates the totals of numeric fields that are right to the control level and places the totals in the corresponding fields of work area.
*————————————————————–* *Data Declaration *————————————————————–*
DATA: gwa_spfli TYPE spfli. DATA: gt_spfli TYPE TABLE OF spfli. SELECT * UP TO 5 ROWS FROM spfli INTO TABLE gt_spfli. LOOP AT gt_spfli INTO gwa_spfli. AT FIRST. WRITE:/ 'Flight Details'. WRITE:/ 'Airline Code' COLOR 5,14 'Connection No.' COLOR 5, 29 'Departure City' COLOR 5, 44 'Arival City' COLOR 5, 58 'Distance' COLOR 5. ULINE. ENDAT. AT NEW carrid. WRITE:/ gwa_spfli-carrid, ' : New Airline'. ULINE. ENDAT. WRITE:/14 gwa_spfli-connid,29 gwa_spfli-cityfrom, 44 gwa_spfli-cityto,58 gwa_spfli-distance. AT END OF carrid. ULINE.SUM.
WRITE:/ gwa_spfli-carrid,58 gwa_spfli-distance. ULINE. ENDAT. AT LAST.SUM.
WRITE:/ 'Total',58 gwa_spfli-distance. WRITE:/ 'End of Loop'. ENDAT. ENDLOOP.Output
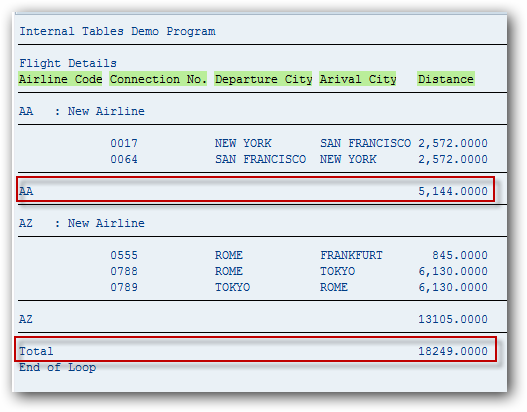
ON CHANGE OF behaves similar to AT NEW. The syntax is as follows.
ON CHANGE OF <control level1> [or <control level2> . .]. [ELSE.] ENDON.*————————————————————–* *Data Declaration *————————————————————–*
DATA: gwa_spfli TYPE spfli. DATA: gt_spfli TYPE TABLE OF spfli. SELECT * UP TO 5 ROWS FROM spfli INTO TABLE gt_spfli. LOOP AT gt_spfli INTO gwa_spfli. AT FIRST. WRITE:/ 'Flight Details'. WRITE:/ 'Airline Code' COLOR 5,14 'Connection No.' COLOR 5, 29 'Departure City' COLOR 5, 44 'Arival City' COLOR 5, 58 'Distance' COLOR 5. ULINE. ENDAT.ON CHANGE OF gwa_spfli-carrid. WRITE:/ gwa_spfli-carrid, ‘ : New Airline’. ULINE. ENDON.
WRITE:/14 gwa_spfli-connid,29 gwa_spfli-cityfrom, 44 gwa_spfli-cityto,58 gwa_spfli-distance. ENDLOOP.Output
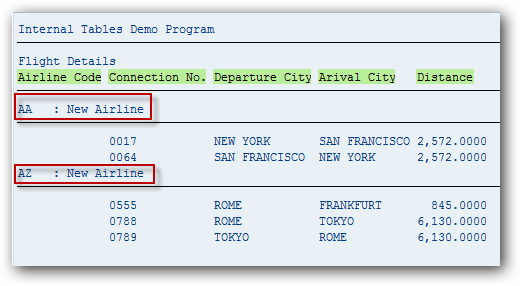
Below table summarizes the differences between AT NEW and ON CHANGE OF statements.
AT NEW | ON CHANGE OF |
---|---|
It can be used only in AT LOOP statement. | It can be used in any loop like SELECT, DO etc.. |
Only one control field can be used. | Multiple control fields separated by OR can be used. |
AT NEW is triggered when a field left to control level changes. | ON CHANGE OF is not triggered when a field left to control level changes. |
Values in the fields to the right of control level contains asterisks and zeros. | Values in the fields to the right of control level contains original values. |
ELSE addition cannot be used. | ELSE addition can be used. |
Changes to work area with AT NEW will be lost. | Changes to work area with ON CHANGE OF will not be lost. |
No comments:
Post a Comment