ABAP Language
ABAP stands for Advanced Business Application Programming. It is a programming language developed by SAP.
ABAP language syntax
- ABAP is not case sensitive.
- Every statement begins with a keyword and ends with a period.( WRITE is the keyword to print on screen )
WRITE 'Hello World!'.
- Chained statements.If consecutive statements have
identical part at the beginning, then ABAP allows you to chain these
statements into a single statement. First write the identical part once
and then place a colon (:). Then write the remaining parts of the
individual statements separated by commas.Normal Statements:
WRITE 'Hello'. WRITE 'ABAP'.
Chained Statement:
WRITE: 'Hello', 'ABAP'.
- Comments.If you want to make the entire line as comment, then enter asterisk (*) at the beginning of the line.
* This is a comment line
If you want to make a part of the line as comment, then enter double quote (“) before the comment.
WRITE 'COMMENT'. "Start of comment
Double click on the SAP icon.
On the logon pad select the system you want to login to and press log on button.
Enter Client, User, password and press enter.
This is SAP easy access screen. All the tools required by ABAP developer is under the node Tools.
What is SAP transaction code?SAP Transaction code is a short cut key attached to a screen. Instead of using SAP easy access menu we can also navigate to a particular screen in SAP by entering the transaction code (T-code for short) in the command field of the standard toolbar.
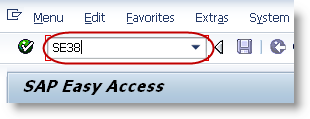
Some of the useful transaction codes for ABAP developers.
T coDe | description |
---|---|
SE11 | ABAP Data Dictionary |
SE16 | Data Browser |
SE37 | Function Builder |
SE38 | ABAP Editor |
SE41 | Menu Painter |
SE51 | Screen Painter |
SE71 | SAP Script Layout |
SE80 | ABAP Workbench |
SE91 | Message Maintenance |
SE93 | Maintain Transaction |
Let us write a “Hello SAP ABAP” program.
Navigate to ABAP editor under Tools node in SAP easy access.
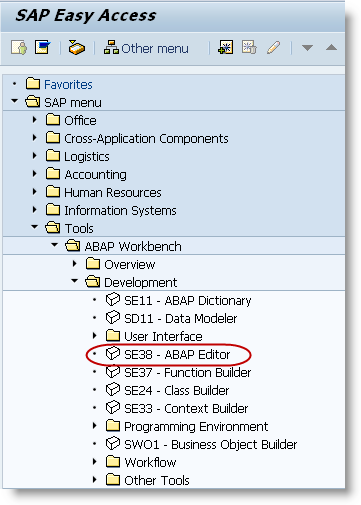
Double click on “ABAP Editor” to open the editor. ABAP editor can also be opened by entering t-code SE38 in the command field.
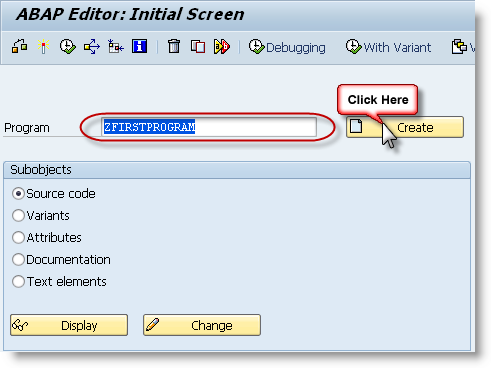
This is the ABAP editor’s initial screen. Enter the name of the program you want to create and press create. All the customer programs must begin with “Y” or “Z”.

In the next popup screen(Program attributes) enter the title for your program, select Executable program as type and press save.
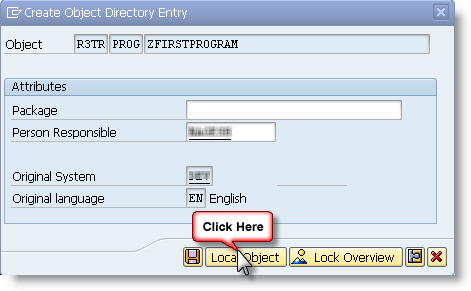
Press Local Object to store the program in the temporary folder.

This is the screen where you can write the ABAP code.
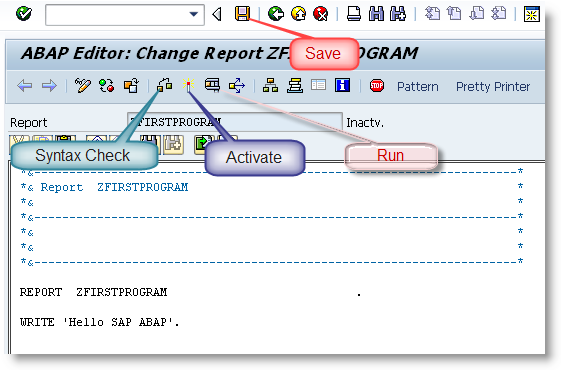
Write the code. Press save, then syntax check( Ctrl + F2 ).

If there are any syntax errors, it ill be displayed at the bottom of the screen as shown above. Correct the errors and again check the syntax.

Successful syntax check message will be displayed in the status bar. Then activate( Ctrl + F3 ) the program.

In the following screen select your program and press continue. Then run(F8) the program.

The output will be displayed as shown above.
ABAP Data Types and Constants
Data Type describes the technical characteristics of a Variable of that type. Data type is just the blue print of a variable.
Predefined ABAP Types
Data Type | Description | Default Length | Default Value |
---|---|---|---|
C | Character | 1 | ‘ ‘ |
N | Numeric | 1 | 0 |
D | Date | 8 | 00000000 |
T | Time | 6 | 000000 |
X | Hexa Decimal | 1 | X’0′ |
I | Integer | 4 | 0 |
P | Packed | 8 | 0 |
F | Float | 8 | 0 |
Use TYPES keyword to define the data types.
TYPES: name(10) TYPE c, length TYPE p DECIMALS 2, counter TYPE i, id(5) TYPE n.Structured data types
Structured data type is grouping of several simple data types under one name.
Use the keywords BEGIN OF and END OF to create a structured data type.
TYPES: BEGIN OF student, id(5) TYPE n, name(10) TYPE c, dob TYPE d, place(10) TYPE c, END OF student.Constants
Constants are used to store a value under a name. We must specify the value when we declare a constant and the value cannot be changed later in the program.
Use CONSTANTS keyword to declare a constant.
CONSTANTS: pi TYPE p DECIMALS 2 VALUE '3.14',
yes TYPE c VALUE 'X'.
ABAP VariablesABAP Variables are instances of data types. Variables are created during program execution and destroyed after program execution.
Use keyword DATA to declare a variable.
DATA: firstname(10) TYPE c, index TYPE i, student_id(5) TYPE n.While declaring a variable we can also refer to an existing variable instead of data type. For that use LIKE instead of TYPE keyword while declaring a variable.
DATA: firstname(10) TYPE c, lastname(10) LIKE firstname. " Observe LIKE keywordStructured Variable
Similar to structured data type, structured variable can be declared using BEGIN OFand END OF keywords.
DATA: BEGIN OF student, id(5) TYPE n, name(10) TYPE c, dob TYPE d, place(10) TYPE c, END OF student.We can also declare a structured variable by referring to an existing structured data type.
TYPES: BEGIN OF address, name(10) TYPE c, street(10) TYPE c, place(10) TYPE c, pincode(6) type n, phone(10) type n, END OF address. Data: house_address type address, office_address like house_address.Each individual field of the structured variable can be accessed using hyphen (-). For example, name field of the house_address structure can be accessed using housing_address-name.
Character is the default data type.
DATA: true. " By default it will take C as data type
ABAP System Variables
ABAP system variables is accessible from all ABAP programs. These fields are filled by the runtime environment. The values in these fields indicate the state of the system at any given point of time.
The complete list of ABAP system variables is found in the SYST table in SAP. Individual fields of the SYST structure can be accessed either using “SYST-” or “SY-”.
WRITE:/ 'ABAP System Variables'. WRITE:/ 'Client : ', sy-mandt. WRITE:/ 'User : ', sy-uname. WRITE:/ 'Date : ', sy-datum. WRITE:/ 'Time : ', sy-uzeit.Output
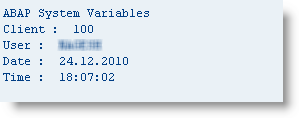
Basic Operations
Assigning values to ABAP variables
Use ‘=’ or MOVE keyword to assign a value to a variable.
DATA: a TYPE i, b TYPE i, c TYPE i, d TYPE i. a = 10. b = a. MOVE 20 TO c. MOVE c TO d. WRITE:/ a, b, c, d.Output

Basic Arithmetic Operations
DATA: a TYPE i, b TYPE i, c TYPE i, d TYPE i. *Using Mathematical Expressions a = 10 + 20. b = 20 - 10. c = 10 * 2. d = 100 / 2. WRITE:/ 'Using Expressions'. WRITE:/ a, b, c, d. *Using Keywords add 10 to a. subtract 5 from b. multiply c by 2. divide d by 2. WRITE:/ 'Using Keywords'. WRITE:/ a, b, c, d.Output
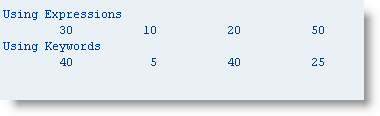
Clear ABAP variables
Use keyword CLEAR to set the variables to default values.
DATA: a TYPE i, b TYPE i. a = 10 + 20. b = 20 - 10. WRITE:/ 'Before Clear'. WRITE:/ a, b. clear: a, b. WRITE:/ 'After Clear'. WRITE:/ a, b.Output
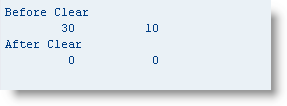
Control Statements
To control the flow of the ABAP program use the following statements.
IF – Branching Conditionally
IF statement – The code between IF and ENDIF is executed only if the condition is true.
DATA: a TYPE i VALUE 10. " We can assign a value in the declaration IF a > 5. WRITE:/ 'Condition True'. ENDIF.Output
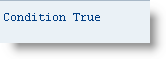
IF-ELSE statement – The code between IF and ELSE is executed if the condition is true, the code between ELSE and ENDIF is executed if the condition is False.
DATA: a TYPE i VALUE 1. IF a > 5. WRITE:/ 'Condition True'. ELSE. WRITE:/ 'Condition False'. ENDIF.Output
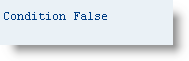
IF-ELSEIF statement – Used to check multiple conditions.
DATA: a TYPE i VALUE 2. IF a > 5. WRITE:/ a, 'Greater Than', 5. ELSEIF a > 4. WRITE:/ a, 'Greater Than', 4. ELSEIF a > 3. WRITE:/ a, 'Greater Than', 3. ELSE. WRITE:/ a, 'Less Than', 3. ENDIF.Output
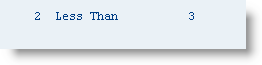
CASE-ENDCASE – Branching based on the content of the variable.
DATA: a TYPE i VALUE 4. CASE a. WHEN 3. WRITE:/ a, 'Equals', 3. WHEN 4. WRITE:/ a, 'Equals', 4. WHEN OTHERS. WRITE:/ 'Not Found'. ENDCASE.Output
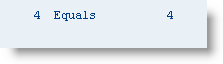
When no condition is met, OTHERS will be executed. OTHERS is not mandatory.
Loops
DO – ENDDO – Unconditional Loop
DO can be used to execute a certain lines of codes specific number of times.
DO 5 TIMES. WRITE sy-index. " SY-INDEX (system variable) - Current loop pass ENDDO.Output

WHILE ENDWHILE – Conditional Loop
WHILE can be used to execute a certain lines of codes as long as the condition is true.
WHILE sy-index < 3. WRITE sy-index. ENDWHILE.Output
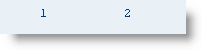
CONTINUE – Terminate a loop pass unconditionally.
After continue the control directly goes to the end statement of the current loop pass ignoring the remaining statements in the current loop pass, starts the next loop pass.
DO 5 TIMES. IF sy-index = 2. CONTINUE. ENDIF. WRITE sy-index. ENDDO.Output

CHECK – Terminate a loop pass conditionally.
If the condition is false, the control directly goes to the end statement of the current loop pass ignoring the remaining statements in the current loop pass, starts the next loop pass.
DO 5 TIMES. CHECK sy-index < 3. WRITE sy-index. ENDDO.Output
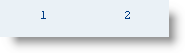
EXIT – Terminate an entire loop pass unconditionally.
After EXIT statement the control goes to the next statement after the end of loop statement.
DO 10 TIMES. IF sy-index = 2. EXIT. ENDIF. WRITE sy-index. ENDDO.Output
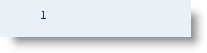
String Operations
CONCATENATE – Combines 2 or more strings into one string.
DATA: s1(10) VALUE 'Hello', s2(10) VALUE 'ABAP', s3(10) VALUE 'World', result1(30), result2(30). CONCATENATE s1 s2 s3 INTO result1. CONCATENATE s1 s2 s3 INTO result2 SEPARATED BY '-'. WRITE / result1. WRITE / result2.Output
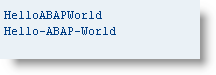
If the the concatenated string fits in the result string, then the system variable sy-subrc is set to 0. If the result has to be truncated then sy-subrc is set to 4.
SPLIT – Splits a string into 2 or more smaller strings.
DATA: s1(10), s2(10), s3(10), source(20) VALUE 'abc-def-ghi'. SPLIT source AT '-' INTO s1 s2 s3. WRITE:/ 'S1 - ', s1. WRITE:/ 'S2 - ', s2. WRITE:/ 'S3 - ', s3.Output
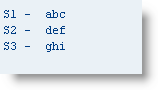
If all target fields are long enough and no target fields has to be truncated then sy-subrc is set to 0, else set to 4.
SEARCH – Searches for a sub string in main string. If found then sy-subrc is set to 0, else set to 4.
DATA: string(30) VALUE 'SAP ABAP Development', str(10) VALUE 'ABAP'. SEARCH string FOR str. IF sy-subrc = 0. WRITE:/ 'Found'. ELSE. WRITE:/ 'Not found'. ENDIF.Output
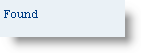
REPLACE – Replaces the sub string with another sub string specified, in the main string. If replaced successfully then sy-subrc is set to 0, else set to 4.
DATA: string(30) VALUE 'SAP ABAP Development', str(10) VALUE 'World'. REPLACE 'Development' WITH str INTO string. WRITE:/ string.Output
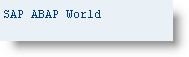
No comments:
Post a Comment